Add SSL to your personal website
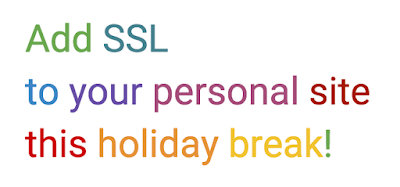
Give yourself a gift this holiday season, and add SSL to your personal site. The web is going secure, and it's time to be part of the solution. This article details how I turned on SSL + custom domains, plus automated deploys, for my personal site for the cost of a domain (which I already had) and $5/year. Read on! Turns out, it's easier (and more affordable!) than you think to add SSL to your website. But first, why bother? There are lots of reasons why you should care about adding SSL: Search engines are preferring SSL New web APIs (like service worker) mandate SSL Users trust SSL Bonus: SSL can help enable HTTP/2 on some servers Your setup will vary, so look for the easiest/shortest path to SSL for your particular site. Everyone has factors they want to optimize for. Here's what I was trying to optimize, as I looked for a solution. I needed a solution that was: Affordable The solution should be very, very affordable. Affordable, in this context, m